TechDesk Overview
TechDesk is built to facilitate seamless interaction between users and administrators within an organization. Users can submit tickets for any issues they encounter, which are then assigned to the relevant department's admin for resolution. The platform supports real-time communication, enabling users and admins to discuss and resolve issues promptly. Additionally, TechDesk includes a chatbot feature, providing immediate assistance and guiding users through common queries even before they start their trial.
Introduction
TechDesk is a powerful helpdesk solution designed to meet the demands of modern organizations. Its comprehensive features, including efficient ticket management, real-time communication, robust admin controls, and AI-driven chatbot support, make it an indispensable tool for enhancing customer service and operational efficiency. By choosing TechDesk, you are investing in a platform that not only addresses your current needs but also evolves with your organization, ensuring sustained excellence in support services.
Key Features
- Ticket Management: Users can quickly submit tickets through a user-friendly interface. Each ticket includes detailed information about the issue, allowing admins to understand and address the problem efficiently.
- Real-Time Communication: TechDesk supports real-time messaging between users and admins, facilitating quick discussions and resolutions. This feature helps in clarifying doubts and providing immediate feedback.
- Admin Management: Admins can manage user accounts, assign roles, and set permissions. This ensures that only authorized personnel have access to specific features and information.
- Chatbot Assistance: The TechDesk chatbot is available 24/7 to assist users with common queries and guide them through the process of submitting tickets. This feature helps in reducing the load on human agents and provides users with immediate answers.
- Security and Compliance: TechDesk employs advanced security measures to protect user data. This includes encryption, secure authentication, and regular security audits.
Benefits
- Improved Efficiency
- Enhanced User Experience
- Scalability
- Cost-Effectiveness
Audience:
This guide is specified for developers to integrate their application with TechDesk
Prerequisites for Using JWT/JWE with Helpdesk System
Before implementing JWT/JWE for secure communication with a Helpdesk system, ensure you have the following in place:
- Registered Application : Your application needs to be registered with the Helpdesk system. This registration process might involve providing information about your application and its intended use. The Helpdesk system has provide specific instructions or an API for registration.
- Secret Key : Upon registration, the Helpdesk system will likely provide you with a secret key. This key is crucial for generating and validating JWT/JWE tokens. Treat this secret key with utmost confidentiality. Do not store it directly in your code or share it publicly.
- JWT/JWE Familiarity : A basic understanding of JWT (JSON Web Token) and JWE (JSON Web Encryption) standards is essential. JWTs represent claims about an entity (e.g., user) and can be used for authorization. JWE builds upon JWT by adding encryption to the payload, protecting sensitive information within the token. You can find resources online or in the Helpdesk system's documentation to learn more about these concepts.
Once you have these prerequisites met, you can proceed with implementing JWT/JWE for secure communication between your application and the Helpdesk system. Refer to the Helpdesk system's documentation for specific instructions and API details.
Register to Techdesk
To integrate our helpdesk features into your applications, you'll need to obtain an Secret key. Follow these steps to get your API key:
- Sign up for a TechDesk account if you haven't already.
This will create an account as well as a company and enable you to use the features of Helpdesk.
- Login to your TechDesk account.
- Navigate to Settings and register a new application.
- Generate an Application Secret key for your application.
- Store your Secret key securely.
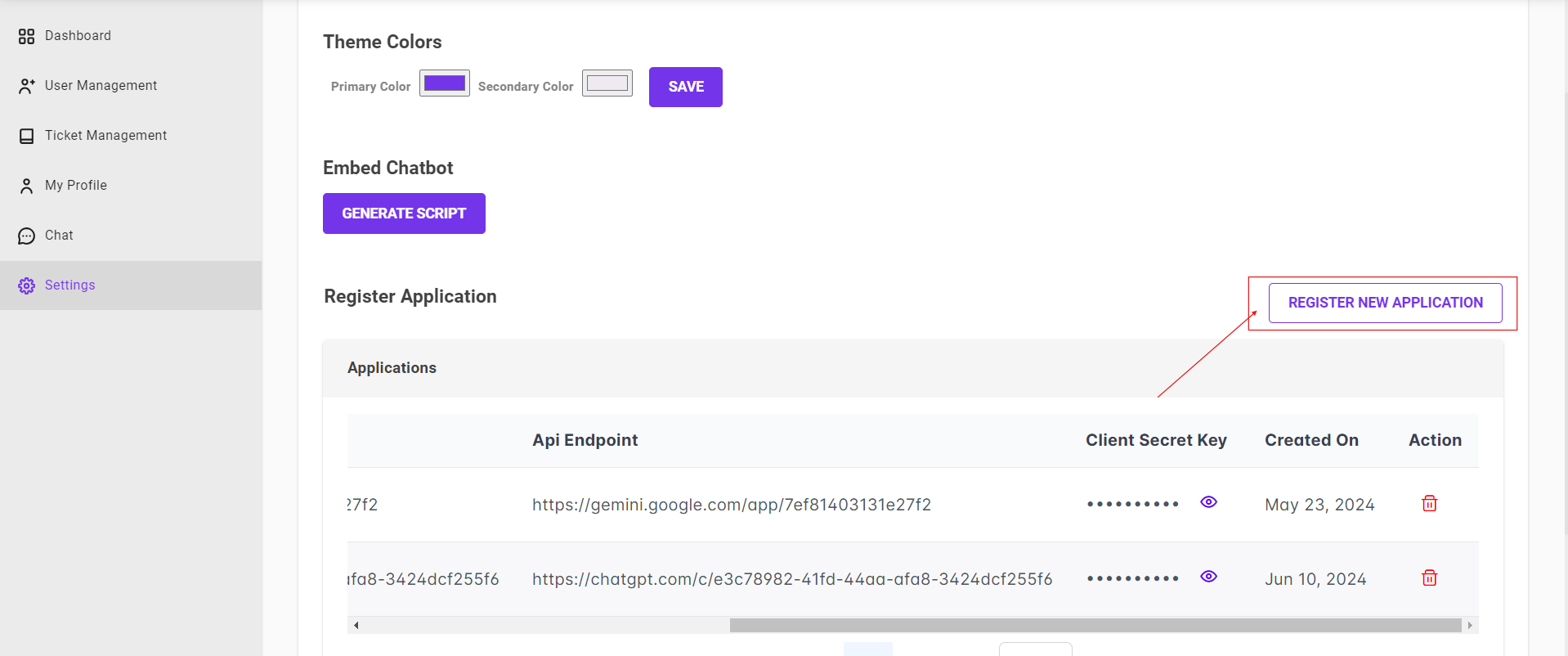
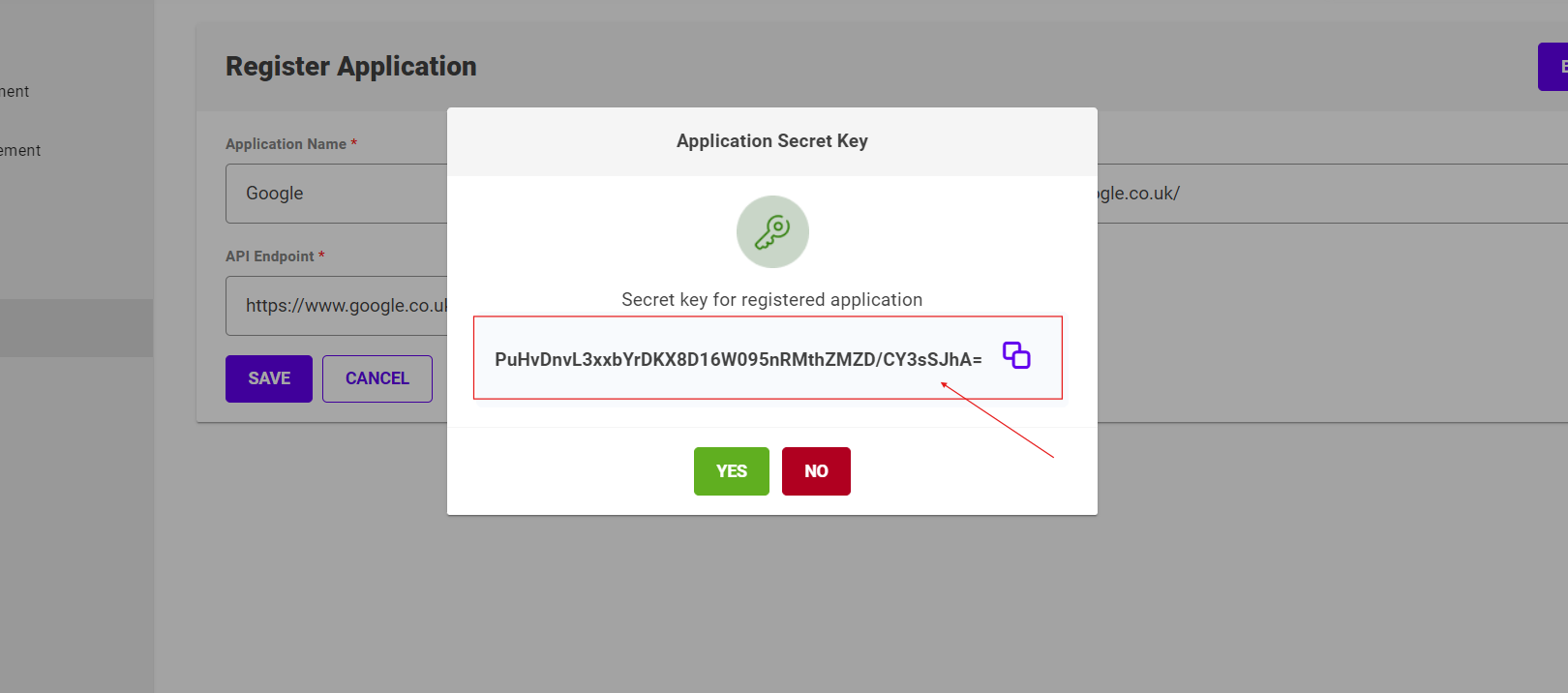
Using your Secret key
With your Secret key in hand, you can now start integrating HelpDesk’s functionalities into your application. Use the key to authenticate API requests, access user data, manage tickets, and more. The API key will be used to generate a JWT token, which includes essential claims such as the user's email and the session start time. This JWT token will be encrypted using JWE (JSON Web Encryption) to ensure the highest level of security.
With your Secret key, you can enable single sign on with Helpdesk and use its services such as ticket management.
Refer to our API documentation for detailed information on how to use your API key to interact with HelpDesk’s services.
JWT (JSON WEB TOKEN)
JWTs are commonly used for authentication in web applications. When a user logs in, the server generates a JWT containing information about the user (payload) and signs it. The client then receives this token and includes it in subsequent requests to authenticate itself. JWTs can also be used for exchanging data securely between parties.
Generate Access Token
- Include User Information : Gather the required information to include in the JWT token:
- User Email
- Session Start Time
- Encode Payload: Create a JSON object containing the user information. For example:
{ "email": "user@example.com", "sessionStartTime": "2024-05-20T15:20:30Z" }
- Encrypt Payload: Use JWE (JSON Web Encryption) to encrypt the payload. This ensures that the token remains secure during transmission.
- Include Token in Requests: Include the encrypted JWT token in the headers of your API requests to authenticate and interact with the service.
- Example Request:
GET https://helpdesk.techbitsolutions.com/externalconnect?accessToken=eyJhbGciOiJBMjU2R0NNS1ciLCJpdiI6Il8zbjdCSmpCTk0yM3hFNzIiLCJ0YWciOiI5c0kyM0hldmdVX3FZMGlWUnd2TFlnIiwiZW5jIjoiQTI1NkdDTSJ9.x4fQfv7JxIRg57-SaKj2pC5Nls9jwQb28vun31KV8JY.F-sSYCpA8T8h0kyD.iEeG-79BqhyIGHHbOOZWAZGKU91MpNafa5ufPdmZRpqZwymHs4xe1hR4F5_ipWU5fyMQogb-mRFwpe-4Of6-5UHsEMhMHnH3BFnm9chAiWt9z-DSJEkQ4XxaUUT-hQQ4SEbaXojvFhoXHJ0Y-Wku0w0MeJHOsZ62EL4aeCC8dWjv_kQOis5k1OM4wAaVatdSA0YijzRuA0ujmRkEu3s.K7wkA50YvMQK3q1Nv1XQUQ&applicationName=ExampleApplicationName
- Query Parameters:
accessToken: A JWT containing user email and session start time. applicationName: The name of your application registered in Helpdesk.
Jose Library for Encryption
The node-jose library simplifies the implementation of JSON Web Encryption (JWE) in Node.js applications, providing robust security mechanisms for protecting sensitive data in transit. It's widely used in applications that require secure communication and data exchange between parties. Adjust the key generation and encryption methods based on your specific security requirements and cryptographic best practices.
- JSON Web Encryption (JWE): node-jose allows you to encrypt sensitive information in JSON format, ensuring confidentiality during transmission over potentially insecure networks.
- Key Management: It provides methods (
createKeyStore
,generate
) to generate cryptographic keys (in this example, RSA keys), which are essential for both encryption and decryption operations. - Encryption and Decryption: The library offers convenient APIs (
JWE.createEncrypt
,JWE.createDecrypt
) to handle JWE operations. You can encrypt data with a public key and decrypt it with the corresponding private key securely. - Compatibility: node-jose supports various algorithms and formats specified by the JSON Web Algorithms (JWA) standard, ensuring interoperability with other compliant libraries and systems.
Generate and Encrypt Token
public string GenerateToken(CipherDataModel dataModel)
{
// Create claims for the JWT Access token
var claims = new[]
{
new Claim("email", dataModel.Email),
new Claim("sessionstarttime", dataModel.SessionStartTime),
};
//Create claims for JWT User Identity Token
var claims = new[]
{
new Claim("email", userResponse.Email),
new Claim("firstName", userResponse.FirstName),
new Claim("lastName", userResponse.LastName),
new Claim("mobilePhone", userResponse.PhoneNumber),
new Claim("userId", userResponse.UserId),
new Claim("userName", userResponse.UserName),
new Claim("userType", userResponse.UserType === "Admin" ? "Admin" : "NormalUser")
};
// Pass the secret key you've generated in helpdesk
var clientSecretKey = _config["ConnectWithHelpdesk:ClientSecretKey"];
var identity = new ClaimsIdentity(claims);
var tokenHandler = new JwtSecurityTokenHandler();
var securityToken = tokenHandler.CreateToken(new SecurityTokenDescriptor
{
Subject = identity,
SigningCredentials = new SigningCredentials(
new SymmetricSecurityKey(Convert.FromBase64String(clientSecretKey)),
SecurityAlgorithms.HmacSha256Signature)
});
var token = tokenHandler.WriteToken(securityToken);
var encryptedToken = EncryptToken(token, clientSecretKey);
return encryptedToken;
}
//Encrypt Token
private string EncryptToken(string token, string clientSecretKey)
{
var payload = JWT.Payload(token);
var encryptedToken = JWT.Encode(payload, Convert.FromBase64String(clientSecretKey), JweAlgorithm.A256GCMKW, JweEncryption.A256GCM);
return encryptedToken;
}
const cors = require('cors');
const jose = require('jose');
const { JWK, JWE } = require('jose');
const genereateHelpdeskToken = async ( { userId }) => {
try {
const user = await knex('users').where('id', userId).first();
//Payload for Access Token
const payload = {
"email": user.email,
"sessionStartTime": new Date().toISOString().split('.')[0] + 'Z',
};
//Payload for User Identity Token
const payload = {
"UserId": userId,
"Email": user.email,
"FirstName": user.first_name,
"LastName": user.last_name,
"Username": user.email,
"UserType": user.role_id === ROLE.SUPER_ADMIN ? "admin" : 'NormalUser',
"MobileNumber": user.phone_number,
}
const clientSecretKey = process.env.HELPDESK_SECRET;
// const secretBuffer = Buffer.from(clientSecretKey, 'base64');
const encryptionKey = {
kty: 'oct',
k: clientSecretKey,
alg: 'A256GCMKW',
use: 'enc',
};
const encryptedToken = await new jose.EncryptJWT(payload)
.setProtectedHeader({ alg: 'A256GCMKW', enc: 'A256GCM' })
.setIssuedAt()
.setExpirationTime(process.env.JWT_EXPIRES_IN)
.encrypt(await jose.importJWK(encryptionKey, 'A256GCMKW'));
return encryptedToken;
} catch (error) {
console.error('Error generating helpdesk token:', error);
throw error;
}
}
//Encrypt Token
const verifyHelpdeskToken = async (token) => {
try {
const clientSecretKey = process.env.HELPDESK_SECRET;
const decryptionKey = {
kty: 'oct',
k: clientSecretKey,
alg: 'A256GCMKW',
use: 'enc',
};
const { payload } = await jose.jwtDecrypt(token, await jose.importJWK(decryptionKey, 'A256GCMKW'));
return payload;
} catch (error) {
console.error('Error verifying helpdesk token:', error);
throw error;
}
};
Why Use JWE (JSON Web Encryption)?
JWE is a security measure that enhances JWT (JSON Web Token) functionality. While JWTs are useful for authorization, JWE adds an extra layer of protection for sensitive information within the token.
JWT vs. JWE for Sensitive Data
Feature | JWT | JWE |
---|---|---|
Function | Claims representation | Claims representation with encrypted payload |
Structure | Header, Payload, Signature | Header, Encrypted Payload, Signature |
Security | Payload not encrypted, signature for integrity/authenticity | Payload encrypted, signature for integrity/authenticity of entire token |
Use Cases | Authorization, user information exchange | Secure transmission of sensitive data |
Why Choose JWE for Sensitive Data?
- Enhanced Security : JWE encrypts the payload, making it unreadable even if intercepted. Only the authorized recipient with the decryption key can access the information.
- Data Protection : Sensitive information like user credentials or financial data requires extra protection. JWE ensures this data remains confidential during transmission.
When Might JWT Be Sufficient?
- Non-Sensitive Claims : If your token primarily contains information used for authorization (e.g., user ID, role), JWT might be enough. These claims are typically not highly sensitive.
- Performance: JWTs are smaller and faster to process compared to JWE tokens. This can be beneficial in performance-critical scenarios.
API Endpoint to generate Token and refresh token
Your application needs to expose an endpoint to handle a POST request which will provide user details to the Helpdesk system and verify the token.
Endpoint Example:
POST https://yourapp.com/api/ConnectWithHelpdesk/GetUserDetails
Helpdesk Request Payload
When interacting with a Helpdesk system using JWT/JWE, the request payload typically includes two key elements:
- Token (JWT/JWE): This is the same token that was initially sent to the Helpdesk system during authentication. It contains information about the user or application making the request. The token format might be JWT (JSON Web Token) or JWE (JSON Web Encryption), depending on the Helpdesk system's security requirements.
- Type: (String) This field specifies the type of operation you want to perform. Common options include:
- GENERATETOKEN: This type indicates a request for a new access token. The Helpdesk system might use the information in the provided JWT/JWE token to verify the user or application and issue a fresh access token if valid.
- REFRESHTOKEN: This type indicates a request to refresh an existing access token. Helpdesk system has implemented refresh tokens for scenarios where access tokens have a limited lifespan. By providing a valid refresh token, you can obtain a new access token without going through the full authentication process again.
The specific format and structure of the request payload might vary depending on the Helpdesk system's API. Always refer to the official documentation for detailed information and examples.
Payload example:
{
token: “eyJhbGciOiJBMjU2R0NNS1ciLCJpdiI6Il8zbjdCSmpCTk0yM3hFNzIiLCJ0YWciOiI5c0kyM0hldmdVX3FZMGlWUnd2TFlnIiwiZW5jIjoiQTI1NkdDTSJ9.x4fQfv7JxIRg57-SaKj2pC5Nls9jwQb28vun31KV8JY.F-sSYCpA8T8h0kyD.iEeG-79BqhyIGHHbOOZWAZGKU91MpNafa5ufPdmZRpqZwymHs4xe1hR4F5_ipWU5fyMQogb-mRFwpe-4Of6-5UHsEMhMHnH3BFnm9chAiWt9z-DSJEkQ4XxaUUT-hQQ4SEbaXojvFhoXHJ0Y-Wku0w0MeJHOsZ62EL4aeCC8dWjv_kQOis5k1OM4wAaVatdSA0YijzRuA0ujmRkEu3s.K7wkA50YvMQK3q1Nv1XQUQ”,
type:”GENERATETOKEN”
}
Helpdesk Response Payload
When your application successfully handles a request from the Helpdesk system, it should respond with a specific JSON structure. This response payload provides information about the request's outcome.
Structure:
{
"status": "int/number",
"message": "string",
"userIdentityToken": "string",
"refreshToken": "string"
}
Elements:
- status (int/number): This field indicates the overall status of the request. Common values include:
- Success (200): The request was processed successfully.
- Bad Request (400): The request contained invalid data or parameters.
- Internal Server Error (500): An unexpected error occurred on your application's side.
- Forbidden (403): The user email associated with the provided token doesn't belong to your application.
- message (string): This field provides a descriptive message explaining the status code. It can help identify the cause of an error or provide more context for a successful response.
- userIdentityToken (string): If the request was successful (status: 200), this field might contain a newly generated JWT (JSON Web Token) as the access token. This token can be used by the Helpdesk system to authorize subsequent requests from your application.
- refreshToken (string - optional): By including a refresh token in the response, can be used to obtain a new access token when the existing one expires.
Payload Example
{
"status": 200,
"message": "Token verified successfully",
"userIdentityToken": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9...",
"refreshToken": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9..."
}
User Identity Token
When sending a `GENERATETOKEN` request payload to a Helpdesk system using JWT/JWE, the concept of `useridentitytoken` might come into play. However, its usage depends on the specific implementation of the Helpdesk system's API.
Important Considerations:
User Identity Token: A `userIdentityToken` is required in the `GENERATETOKEN` request. When it expires, the helpdesk system sends a request with the refresh token (only if the integrating application has provided a valid refresh token and it has not expired yet). If the refresh token has expired, the helpdesk system will send the user identity token to obtain a new user identity token and refresh token.
- Security : If your application uses an external authentication system, ensure the `userIdentityToken` is obtained securely and not exposed in transit or logs.
JWT Payload for accessToken:
{
"UserId": "12345",
"Email": "user@example.com",
"FirstName": "John",
"LastName": "Doe",
"Username": "johndoe",
"UserType": "NormalUser",
"MobileNumber": "123-456-7890"
}
JWT Payload for accessToken description:
- UserId (from Integrating application): This field can be used to identify the user within your your system. It's helpful for associating Helpdesk actions with specific users in your CRM.
- Email: The user's email address. The Helpdesk system enforces email uniqueness, so if you encounter duplicate emails, contact the Helpdesk admin for guidance.
- Username: The user's username within your system. Similar to email, usernames are unique in the Helpdesk system. Contact the Helpdesk admin if you have duplicate usernames.
- First Name : The user's first name within your system. Similar to email, first names are unique in the Helpdesk system.
- Last Name - (optional) : The user's last name within your system. Similar to email, last names are unique in the Helpdesk system.
- UserType: This field specifies the user's role within the Helpdesk system. Currently, two user types are supported:
- Admin: Grants administrator privileges within the Helpdesk system.
- Normaluser: Grants standard user privileges within the Helpdesk system.
- Mobile Phone - (optional) : The user's phone number within your system. Similar to email, phone numbers are unique in the Helpdesk system.
Important Considerations:
- Security : Ensure that the user information included in the JWT/JWE claims is protected. Consider using JWE (JSON Web Encryption) if sensitive user data like email needs extra protection during transmission.
Refresh Tokens
When a token is expired, techdesk will request for new access token by sending the user identity token as a request payload and in response get back a new user identity token and refresh token.
To avoid forcing users to log in again every time their access token expires, a refresh token is used. A refresh token has a longer lifespan and can be used to request a new access token without requiring the user to re-authenticate.
Token Expiration:
JWTs often include an expiration time (exp claim) to ensure they are only valid for a limited period. Once this period has passed, the token is considered expired, and the user must obtain a new one to continue accessing protected resources.
How it Works:
Initial Authentication:
The user logs in and receives an user identity token and a refresh token.
The user identity token is used to authenticate requests to protected resources.
Token Expiration:
When the access token expires (determined by the exp
claim), the client detects the expiration and initiates a token renewal process.
Requesting a New User Identity Token:
The client sends the refresh token to the authentication server to request a new user identity token.
This request typically includes the refresh token and possibly the user identity token.
Server Response:
The authentication server validates the refresh token.
If valid, the server issues a new user identity token (and possibly a new refresh token) in response.
Using the New Token:
The client receives the new user identity token and uses it for subsequent requests to protected resources.
Token Validation
Token validation is a crucial process that ensures the authenticity and integrity of the payload data transmitted between systems. This involves verifying that the token has been properly signed by the issuing authority and that it has not been tampered with or expired.
- Signature Verification: The token's signature is checked against the public key or secret key used by the issuer. This ensures that the token was indeed issued by a trusted authority and has not been altered.
- Expiration Check: Tokens typically include an expiration time. Validation involves checking the current time against this expiration time to ensure the token is still valid and has not expired.
- Issuer and Audience Validation: The token should contain claims specifying the intended audience and the issuer. These claims are validated to ensure the token is being used by the correct recipient and was issued by the expected source.
- Claims Integrity: The payload of the token, often containing user information and permissions, is checked to ensure it has not been modified. This maintains the integrity of the data being transmitted.
Endpoint exposed by Helpdesk to validate the token
POST https://helpdesk.techbitsolutions.com/api/ExternalAuthorization/ValidateToken
Request for validating tokens
This endpoint requires three parameters:
- Token: The access token that needs to be validated.
- SecretKey: The secret key used to validate the token's authenticity.
- Type: Specifies the type of token. In this case, it should be "accessToken".
To check the endpoint POSTMAN can be used
Access Token Validation
For an access token, the important claims to verify are:
- Session Start Time: The time when the session started, to ensure it is within the valid time frame.
- Email: The email address associated with the token, to confirm the identity of the user.
- Expiry: The expiration time of the token, to ensure it has not expired.
User Identity Token Validation
For a user identity token, the important claims to verify are:
- Username: The username associated with the token, to confirm the user's identity.
- First Name: The first name of the user, to validate the user information.
- User Type: The type of user (e.g., admin or normal user), to check the user's role.
- UserId: The unique identifier of the user, to ensure the token corresponds to the correct user.
- Email: The email address associated with the token, to confirm the identity of the user.
- Expiry: The expiration time of the token, to ensure it has not expired.
Invalid Token
If the token provided is not valid, the server will respond with a status code of 400 and a message indicating that the token is invalid. This can occur if the token has been tampered with, is malformed, or fails verification.
{
"status": 400,
"message": "Invalid token"
}
Token Expired
When the token has passed its expiration time, the server will respond with a status code of 400 and a message indicating that the token has expired. This indicates that the client needs to use the refresh token to obtain a new access token.
{
"status": 400,
"message": "Token expired"
}
Server Errors
If the Helpdesk system encounters an error while processing the token, it will respond with an appropriate HTTP status code and a message describing the error. These errors could be due to server-side issues, such as database connectivity problems or other internal errors.
{
"status": 500,
"message": "Internal server error"
}